Welcome back, C# enthusiasts! Today, we’re diving into an exciting topic: using LINQ for file system operations. LINQ, or Language Integrated Query, is a fantastic tool for querying data, and it’s not just limited to databases. We can leverage LINQ to perform efficient, non-destructive queries on files and directories. This approach allows us to write clean, expressive code that’s both powerful and easy to read.
In this blog post, we’ll explore some practical examples that demonstrate the true power of LINQ in file system operations. Let’s get started!
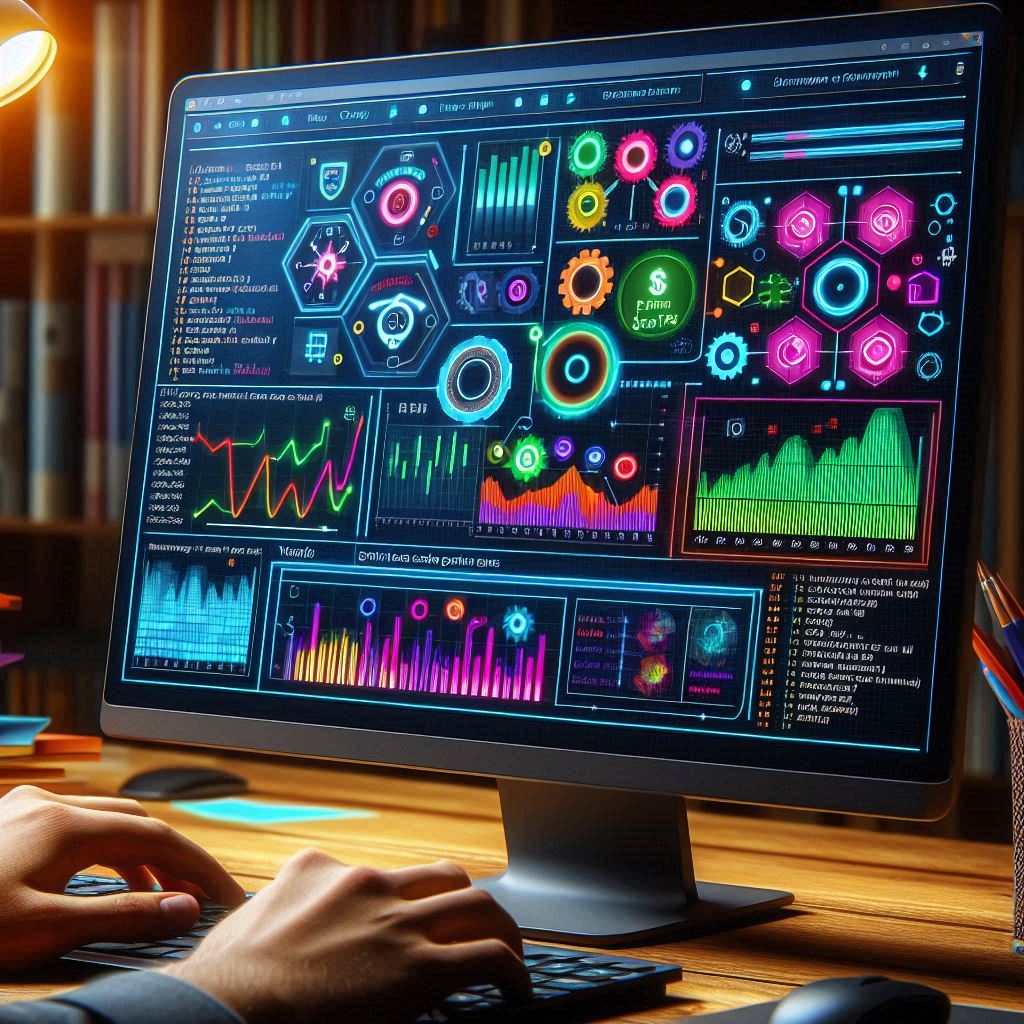
Finding Files with Specific Attributes
Imagine you need to find all text files in a directory tree and identify the newest one. Here’s how you can do it with LINQ extension methods:
string startFolder = @"C:\Temp";
var fileList = new DirectoryInfo(startFolder).GetFiles("*.*", SearchOption.AllDirectories);
var textFiles = fileList
.Where(file => file.Extension == ".txt")
.OrderBy(file => file.Name);
var newestFile = textFiles.OrderByDescending(f => f.CreationTime).First();
Console.WriteLine($"The newest .txt file is {newestFile.FullName}. Created on: {newestFile.CreationTime}");
This code snippet elegantly filters, sorts, and selects files, showcasing the expressive power of LINQ.
Grouping Files by Extension
LINQ really shines when it comes to grouping and sorting. Check out this example:
var groupedFiles = fileList
.GroupBy(file => file.Extension.ToLower())
.OrderBy(group => group.Count())
.ThenBy(group => group.Key);
foreach (var group in groupedFiles.Take(5))
{
Console.WriteLine($"Extension: {group.Key}");
foreach (var file in group.Take(3))
{
Console.WriteLine($"\t{file.Name}");
}
}
This query groups files by extension, orders them and then displays the top 5 groups with 3 files each.
Calculating Total File Size
Need to know the total size of files in a directory? LINQ makes it a breeze:
var totalSize = fileList
.Select(file => new FileInfo(file.FullName).Length)
.Sum();
Console.WriteLine($"Total size of all files: {totalSize}");
For total size, you can also use the following:
totalSize = fileList.Sum(f => f.Length);
This concise query calculates the total size of all files in the directory tree.
Advanced Techniques: Finding Duplicate Files
Now, let’s look at an advanced technique: finding duplicate files across directories. LINQ can help you identify duplicate files easily:
var duplicates = fileList
.GroupBy(file => new { file.Name, file.Length })
.Where(group => group.Count() > 1);
foreach (var group in duplicates)
{
Console.WriteLine($"Duplicate found: {group.Key.Name} ({group.Key.Length} bytes)");
foreach (var file in group)
{
Console.WriteLine($"\t{file.FullName}");
}
}
This query groups files by name and size and then selects groups with more than one file.
Conclusion
As you can see, LINQ transforms complex file system operations into elegant, readable code. It’s a powerful tool that every C# developer should master. By using LINQ, you can write clean, expressive code that’s both powerful and easy to read.
In this blog post, we’ve covered:
- Finding files with specific attributes
- Grouping files by extension
- Calculating the total file size
- Finding duplicate files
I hope you found this post helpful and that it inspires you to explore more advanced LINQ techniques for file system manipulation. If you have any questions or comments, feel free to leave them below. And don’t forget to share this post with your fellow C# developers!
This coding session is covered in my YouTube video here.