In the ever-evolving landscape of software development, leveraging advanced tools can significantly enhance productivity and code quality. One such tool is ChatGPT, an AI language model developed by OpenAI, which can assist C# developers in various aspects of coding.
In this blog post, we’ll explore practical ways to integrate ChatGPT into your C# development workflow to debug errors, optimize performance, generate boilerplate code, and write unit tests.
How ChatGPT Can Assist C# Developers in Code
ChatGPT offers a range of functionalities that can be beneficial for C# developers:
- Explaining C# Concepts: Clarifies complex programming concepts and syntax.
- Debugging Errors: Helps identify and resolve coding errors.
- Optimizing Performance: Suggests improvements for more efficient code.
- Generating Boilerplate Code: Provides templates for common coding structures.
- Writing Unit Tests: Assists in creating tests to ensure code reliability.
By incorporating ChatGPT into your workflow, you can streamline development processes and enhance code quality.
Practical Examples of using ChatGPT in C# Code
Let’s delve into specific scenarios where ChatGPT can be a valuable asset in C# development.
1. Debugging Errors
Encountering an “Index out of range exception” is a common issue in C#. Consider the following code snippet:
int[] numbers = {1, 2, 3, 4, 5};
Console.WriteLine(numbers[5]); // Index out of range exception
By inputting this code into ChatGPT and describing the error, the AI can explain that arrays in C# are zero-based, meaning the valid indices for the numbers
array are 0 through 4. Accessing numbers[5]
exceeds this range, causing the exception. ChatGPT might suggest checking the array’s length before accessing an index to prevent such errors.
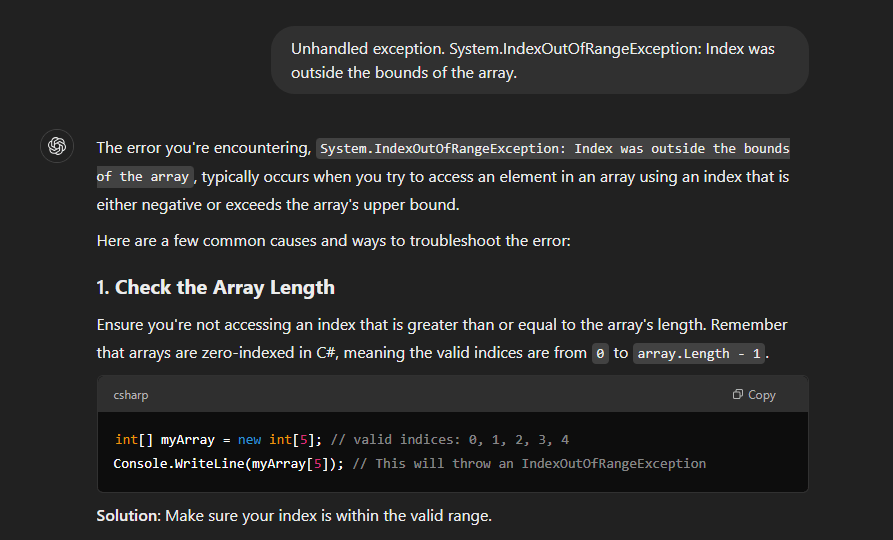
2. Optimizing Code
Consider a scenario where you have a loop to calculate the sum of an array:
int sum = 0;
for (int i = 0; i < numbers.Length; i++)
{
sum += numbers[i];
}
Console.WriteLine(sum);
ChatGPT can suggest a more concise and efficient approach using LINQ:
int sum = numbers.Sum();
Console.WriteLine(sum);
This method enhances code readability and leverages the power of LINQ for efficient data manipulation.
3. Generating Boilerplate Code
When you need to create a basic class structure, ChatGPT can expedite the process. For instance, you can prompt:
“Generate a C# class for a Car
with properties for Make
, Model
, and Year
, and a method to display details.”
ChatGPT can provide the following code:
public class Car
{
// Properties for Make, Model, and Year
public string Make { get; set; }
public string Model { get; set; }
public int Year { get; set; }
// Constructor to initialize a Car object
public Car(string make, string model, int year)
{
Make = make;
Model = model;
Year = year;
}
// Method to display the car details
public void DisplayDetails()
{
Console.WriteLine($"Car Details: {Year} {Make} {Model}");
}
}
This saves time and ensures consistency in your codebase.
4. Writing Unit Tests
Writing unit tests is crucial for maintaining code reliability. ChatGPT can assist by generating test methods. For example:
“write a NUnit test case for Car
class DisplayDetails
method, consider all possible boundary conditions and exceptions”
ChatGPT might generate:
using NUnit.Framework;
using System;
using System.IO;
[TestFixture]
public class CarTests
{
// Helper method to capture console output
private string CaptureConsoleOutput(Action action)
{
var consoleOutput = new StringWriter();
Console.SetOut(consoleOutput);
action();
return consoleOutput.ToString().Trim(); // Trim to remove extra newlines
}
[Test]
public void DisplayDetails_ValidInput_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("Toyota", "Corolla", 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020 Toyota Corolla", output);
}
[Test]
public void DisplayDetails_EmptyMake_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("", "Corolla", 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020 Corolla", output); // Empty make
}
[Test]
public void DisplayDetails_EmptyModel_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("Toyota", "", 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020 Toyota", output); // Empty model
}
[Test]
public void DisplayDetails_EmptyMakeAndModel_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("", "", 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020", output); // Both empty
}
[Test]
public void DisplayDetails_InvalidYear_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("Toyota", "Corolla", 3000); // Future year
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 3000 Toyota Corolla", output); // Handles any valid integer year
}
[Test]
public void DisplayDetails_NullMake_ReturnsCorrectOutput()
{
// Arrange
var car = new Car(null, "Corolla", 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020 null Corolla", output); // Null make
}
[Test]
public void DisplayDetails_NullModel_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("Toyota", null, 2020);
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 2020 Toyota null", output); // Null model
}
// Additional test cases could include validation for edge year values like 0 or negative years.
[Test]
public void DisplayDetails_ZeroYear_ReturnsCorrectOutput()
{
// Arrange
var car = new Car("Toyota", "Corolla", 0); // Invalid year
// Act
var output = CaptureConsoleOutput(() => car.DisplayDetails());
// Assert
Assert.AreEqual("Car Details: 0 Toyota Corolla", output); // Handles zero year
}
}
This provides a solid foundation for your unit tests, which you can further customize as needed.
Best Practices for Using ChatGPT in C# Code
While ChatGPT is a powerful tool, it’s essential to use it judiciously:
- Review Generated Code: Always review and test the code provided by ChatGPT to ensure it meets your application’s requirements and adheres to best practices.
- Understand Suggestions: Use ChatGPT’s suggestions as learning opportunities to deepen your understanding of C# concepts.
- Maintain Security: Be cautious about sharing sensitive code or data with AI tools.
By following these practices, you can effectively integrate ChatGPT into your development process, enhancing both efficiency and code quality.
Conclusion
Integrating ChatGPT into your C# development workflow can be a game-changer. From debugging and optimization to code generation and testing, ChatGPT offers valuable assistance that can streamline your coding process. Embrace this AI-powered tool to elevate your coding experience and produce high-quality C# applications.
You can watch this session on YouTube here.
Note: While ChatGPT is a powerful assistant, always ensure that you validate and test the code it generates to align with your specific project requirements.