What is .NET Core
.NET Core or .NET 5 is an implementation of the .NET Framework, which is built in the open (OSS) from day 1 and it runs on all major platforms (Windows, Linux, and Mac).
We can use .NET Core for building Web applications and Console applications. We can use C#, F#, or VB.Net for writing applications in .NET Core/.NET 5.0. The .NET 5.0 uses .NET Standards as a base set of APIs.
In the last few years, the .NET Core framework has evolved significantly. The current version of the framework is .NET 5.0 and is the version that will be supported going forward.
For the legacy .NET Framework, there will not be any new development and will only be patched for any security concerns.
In this blog, I am going to create a .NET 5.0 Console application. I am going to use the .NET 5.0 CLI for creating the application to demonstrate how easy it is to create a .NET 5.0 application especially using the CLI.
I am using a Window 10 PC for the development.
What is .NET 5 compared to .NET Core
Firstly, .NET 5 is the successor version of .NET Core 3.2. It is the version of the Framework that will be supported going forward.
Secondly, just as .NET Core was, The .NET 5.0 framework is the cross-platform and open-source latest version of .NET.
And finally, the .NET 5 Framework is all the features of .NET Core plus some more.
Installation of .NET Core
To install the .NET 5.0 framework (the latest .NET Core framework), firstly, either we can download and install Visual Studio 2019.
Or secondly, we can just download and install the .NET 5.0 SDK. I am going to just download the .NET 5.0 SDK from https://www.microsoft.com/net/download/windows.
On the download page, from the latest version “SDK 5.0.101”, I will go down and select the x64 link for Windows, which downloaded the SDK on my PC.
The download size is 144 MB. After the download is complete I will install the SDK using the one-click Install button. The installation takes about a couple of minutes to complete.
After the installation is complete, I will open up the command prompt and type dotnet. This command will show the following output on the screen indicating the installation was successful.
C:\>dotnet
Usage: dotnet [options]
Usage: dotnet [path-to-application]
Options:
-h|--help Display help.
--info Display .NET information.
--list-sdks Display the installed SDKs.
--list-runtimes Display the installed runtimes.
path-to-application:
The path to an application .dll file to execute.
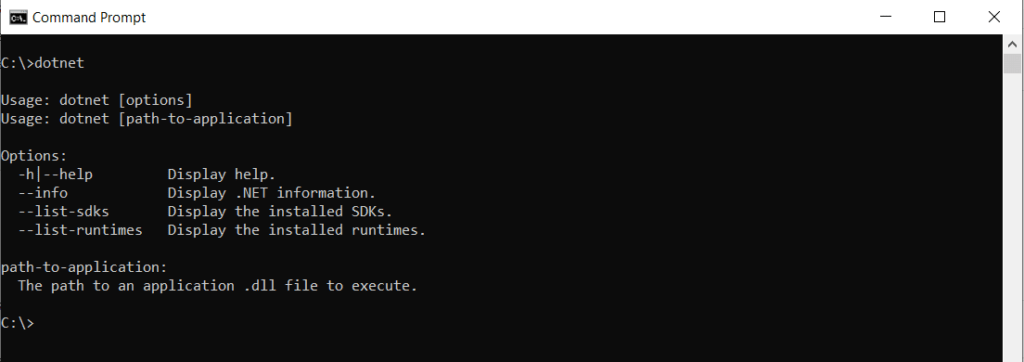
Project Creation
Since the SDK installation is complete, now I am going to create a .NET 5.0 Console application.
For that firstly, I will create a new folder in my C drive named “code”.
Secondly, I will navigate to the “code” folder.
C:\mkdir code
C:\cd code
Thirdly, after the first two steps, I will create a new console application using the following command:dotnet new console -o test1
Finally, I will navigate to the newly created project folder.
cd test1
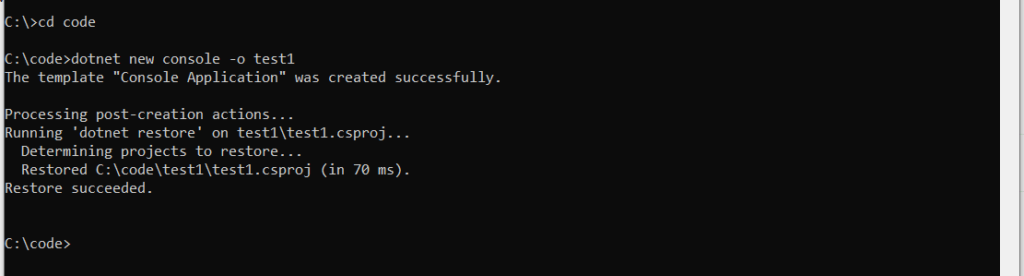
Code Inspection
After the previous steps, a new console application will create inside of the “Code” folder with the name of “test1”.
This “test1” folder contains the following files:
- Firstly, a C# file with the name of Program.cs
- Secondly, a C# project file with the name of test1.csproj
- Finally, a folder named “obj”
These are the usual items we will see when we create a new C# project with Visual Studio as well.
Next, I will open the Program.cs file from the command prompt using the following command:
C:\notepad Program.cs
This will open the Program.cs in the notepad. The follwing is the content of the Program.cs file:
using System;
namespace test1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
As you can see above, the content of the Program.cs class is very simple.
Firstly, It has the Program class containing the code.
Secondly, inside of the the Program class, we have the Main method, which will be invoked by the framework when the application starts. In other word, this is the entry point of the application.
Finally, inside of the Main method, we have a Console.WriteLine statement, which prints out the statement “Hello World!”.
Running the application
Before I can run the application, firstly, I will go ahead and change the text “Hello World!” to “Hello World to .NET Core Lovers!”. Secondly, I will save the file.
using System;
namespace test1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World to .NET Core Lovers!");
}
}
}
Finally, I will run the following command in the command prompt to run the application:
C:\dotnet run
And once I run the above command, it will print the following output in the console:Hello World to .NET Core Lovers!

Conclusion
Firstly, I am impressed by the simplicity of the .Net Core. This is just the start for me. I will start with a real-life project and share my experiences!
Secondly, the framework has got a lot of traction in the open source community.
Thirdly, in the Stack overflow developer survey, it has scored one of the most loved frameworks in last couple of years.
I have a video with the steps followed for creating the console application, here is the link to the YouTube video here.
References
Firstly, for .Net Core SDK Download you can visit the Link: https://www.microsoft.com/net/download/windows
Secondly, for what is .Net, you can visit the Link: https://www.microsoft.com/net/learn/what-is-dotnet
Finally, for .Net Core Getting Started, you can visit the Link: https://www.microsoft.com/net/learn/get-started/windows