In this blog post, I am going to talk about AWS Lambda. AWS Lambda is a serverless compute service provided by Amazon Web Services. When we use serverless features, we do not have to worry about managing the infrastructure, managing how to scale out or scale down. And that is the biggest value add for the serverless functions or any other serverless feature provided by AWS.
In today’s blog post I am going to focus only on lambda. But in subsequent blog posts, I am going to talk about other serverless features like DynamoDB, SQS, SNS, etc.
But with any serverless features, it comes with a price. Lambda is usually costlier compared to using compute like EC2 if the EC2 is optimized properly. The EC2 optimization is the key here because based on the use case using AWS Lambda might be a better option.
Where we should use AWS Lambda
Just like any other features, serverless also has a lot of hypes and that is why it is very important to understand where to use it.
Just like any other technology or programming language, everything has its own place, and architecturally not everything fits everywhere. So we have to be very cautious choosing AWS Lambda or serverless computing.
The first and the most important thing to keep in mind is that Lambda is best suited for smaller functions. Not even a microservice, more like nano services. It is a very small unit of computing that you want to execute.
For example, sending an email or sending a text message. Or running a computation to deliver something, but a single responsibility function. That is how lambda should be designed.
Second thing to keep in mind, is that Lambda integrates extremely well with other aws serverless services like SQS, SNS, etc.
Finally, whenever you use Lambda the function, time should be minimal or the execution time for the function should be minimal. Since you pay for the compute time used. So it does not make sense to run highly time-consuming operations in a Lambda. And to be honest that is not what its main purpose is.
Where we should not use AWS Lambda
Where not to use AWS Lambda? As I mentioned earlier we should not use it for continuously running processes and long-running processes. So if you have an application that has a listener to maybe a queue, but it gets millions of messages a day and it does a lot of processing. In that kind of situation, Lambda is not the right answer.
The other situation where we should not use Lambda is if you have a process that does a map/reduce job and gets a lot of data and creates some aggregations. And finally saves the data into a database or a table, which is also not a good use case for Lambda. Because it will take a lot of time it’s a long running process.
We should not use lambda where we have a very large footprint.
And then we should not use Lambda for places where we have the requirement of high frequency or low latency. The reason for that is the Lambda functions are loaded only when it is requested.
And finally, complex business logic with multiple transactions should not be in Lambda. Lambda function is not meant for that.
Creating a Lambda Function
Now that we discussed what is AWS Lambda and where to use or not to use it. Let us go and see how to create a new Lambda function.
I am going to use aws cli for creating a new Lambda function.
Firstly, you will need to create an AWS account. You can create an account with AWS for free. It needs a credit card to validate but it does not charge you anything.
And you can get a lot of features free for a year. But there are features which are always free. Things like Lambda there are one million function calls that you can make for a year.
Using AWS CLI
Firstly we will need to install the AWS CLI. The link for downloading and installing AWS CLI is here.
After AWS CLI is installed, we will need to use the command aws configure
and provide a profile name. And then once we do that, it is going to ask for AWS key and secret, so we will provide that. Once we provide that, our PC will configure itself to use the AWS CLI.
The next thing we will need is AWS Lambda tools. For that we will use the following command:
dotnet tool install -g Amazon.Lambda.Tools
This will install the Lambda tools globally.
Next, we will install templates for the AWS Lambda function. And for that we will use the following command:
dotnet new --install Amazon.Lambda.Templates::5.2.0
I am going to create the AWS Lambda function using the .NET CLI and AWS Lambda Tools. It will be very easy to do that. I am going to use the following command for that:
dotnet new lambda.EmptyFunction --name LambdaDemo
The above command will create a folder in the file system with the name LambdaDemo and inside the folder, it will have one folder src for Lambda code and another folder test for unit tests.
The code
Inside the new project created, there will be a single class Function. It will have a single function named FunctionHandler.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Amazon.Lambda.Core;
// Assembly attribute to enable the Lambda function's JSON input to be converted into a .NET class.
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.SystemTextJson.DefaultLambdaJsonSerializer))]
namespace MyFunction
{
public class Function
{
/// <summary>
/// A simple function that takes a string and does a ToUpper
/// </summary>
/// <param name="input"></param>
/// <param name="context"></param>
/// <returns></returns>
public string FunctionHandler(string input, ILambdaContext context)
{
return input?.ToUpper();
}
}
}
And as you can see this function is just converting the incoming string input to upper case. The ILambdaContext can be used to get access to the context and use functionality like logging.
Deploying the function to AWS
Deploying the function in AWS is very straightforward. We can use the following command to deploy the function:
dotnet lambda deploy-function LambdaDemo --profile personal --region us-east-2
Once deployed, this is how the function will look like:
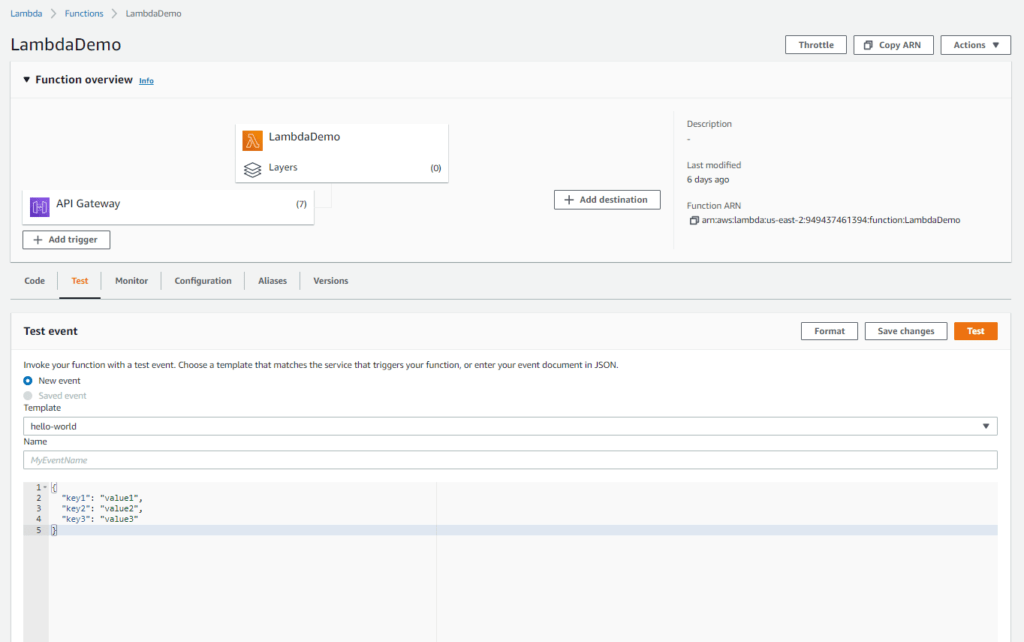
Using the Test tab, we can test our lambda. And once tested the test result in the Test tab and the logs will show in the AWS Cloudwatch logs.
The YouTube video for this blog is available here.