In my last blog post https://dotnetcorecentral.com/blog/dependency-injection-in-net-core-console-application/, I have explored using Dependency Injection in .NET Core console application. But, in this blog post, I will explore using Autofac as the Dependency Injection container for .NET Core Console application.
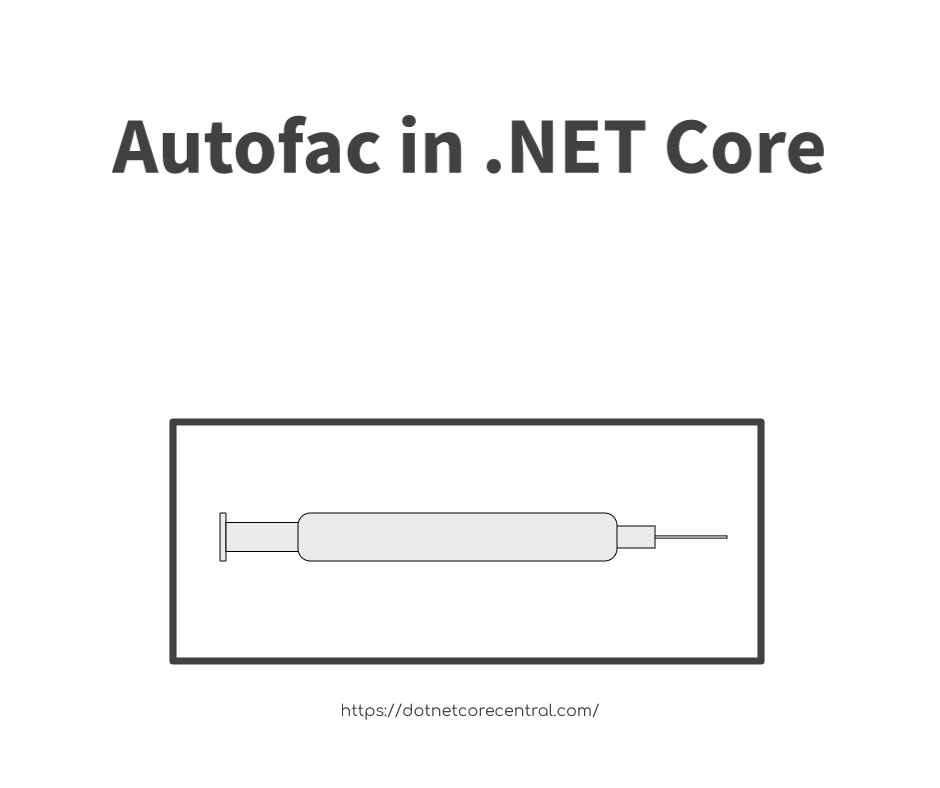
What is Autofac
Autofac is an Inversion Of Control (IoC) container for .NET Framework and .NET Core. I have been using it for more than five years now. It is really easy to pick up. Above all, it integrates with .NET Core works flawlessly. You can visit their website at https://autofac.org/.
Adding NuGet packages
Firstly, I will open the console application I have created for Docker demo (please refer to my blog: https://dotnetcorecentral.com/blog/docker-container-for-net-core/).
Secondly, I will open the Nuget Package Manager for the project Docker.Demo
.
Thirdly, I will add the NuGet package Autofac.Extensions.DependencyInjection
to the project.

Updating ContainerConfiguration class
I will update the Configure
method of the ContainerConfiguration
class with the following changes:
Firstly, I will create an instance of ContainerBuilder
class provided by Autofac.
Secondly, I will call the Populate
method of the ContainerBuilder
passing the instance of ServiceCollection
. This is a very important step, as it hooks up the Autofac IoC container to the .NET Core Dependency injection framework.
Thirdly, I will add all the registration to the ContainerBuilder
instance.
Finally, I will build the ContainerBuilder
instance and return a new instance of AutofacServiceProvider
, passing the built ContainerBuilder
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | using Autofac; using Autofac.Extensions.DependencyInjection; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Logging; using System; namespace Docker.Demo { internal static class ContainerConfiguration { public static IServiceProvider Configure() { var serviceCollection = new ServiceCollection(); serviceCollection.AddLogging(l => l.AddConsole()) .Configure<LoggerFilterOptions>(c => c.MinLevel = LogLevel.Trace); var containerBuilder = new ContainerBuilder(); containerBuilder.Populate(serviceCollection); containerBuilder.RegisterType<PrintSettingsProvider>().As<IPrintSettingsProvider>().SingleInstance(); containerBuilder.RegisterType<ConsolePrinter>().As<IConsolePrinter>().SingleInstance(); containerBuilder.RegisterType<ContinuousRunningProcessor>().SingleInstance(); var container = containerBuilder.Build(); return new AutofacServiceProvider(container); } } } |
Frequently used ContainerBuilder methods
Following are the methods, which will be frequently used:
- RegisterInstance – Register an object. E.g,
builder.RegisterInstance(new SomeType())
.As<ISomeInterface>(); - RegisterType<T> – Register a type. E.g,
builder.RegisterType<SomeType>().As<ISOmeInterface>();
- SingleInstance – Only one instance of the type throughout the application. E.g.,
builder.RegisterType<SomeType>().As<ISOmeInterface>().SingleInstance();
- InstancePerDependency – New instance on every request. E.g.,
builder.RegisterType<SomeType>().As<ISOmeInterface>().InstancePerDependency();
- Named – A named instance; the name will be used to retrieve the instance from the container. Very useful if the same interface is implemented by two classes. E.g,
builder.RegisterType<SomeType>().As<ISOmeInterface>().SingleInstance().Named<string>("SomeName");
.
The output of the application
Now, I will run the application, and the response would be as expected.
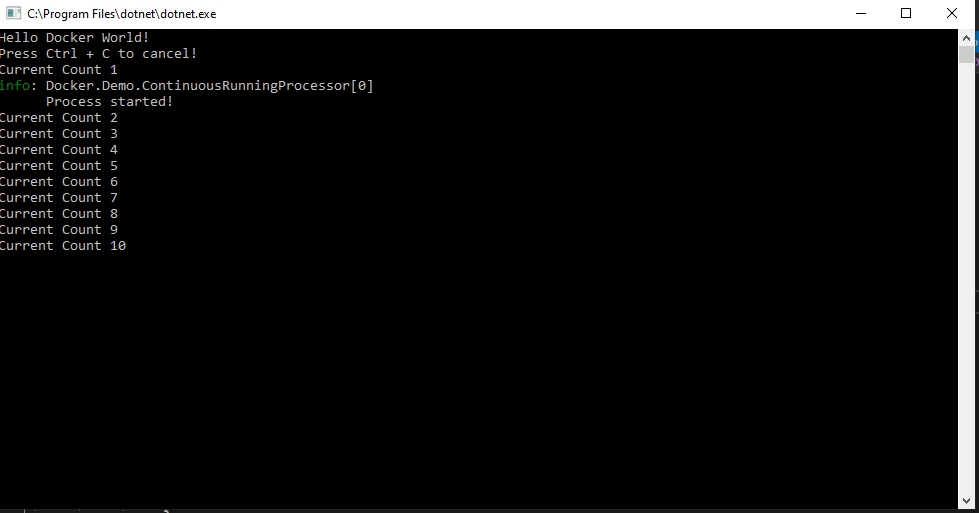
YouTube Video link: https://youtu.be/hNPAkDyqZ_I
Link to the source code: https://github.com/choudhurynirjhar/docker-demo