As a C# developer who has battled with collection manipulation for over a decade, I can’t count how many times I’ve seen developers write complex nested loops when LINQ set operations could have solved their problems in a single line. Today, I’m going to share these powerful yet underutilized LINQ features that have saved me countless hours of coding.
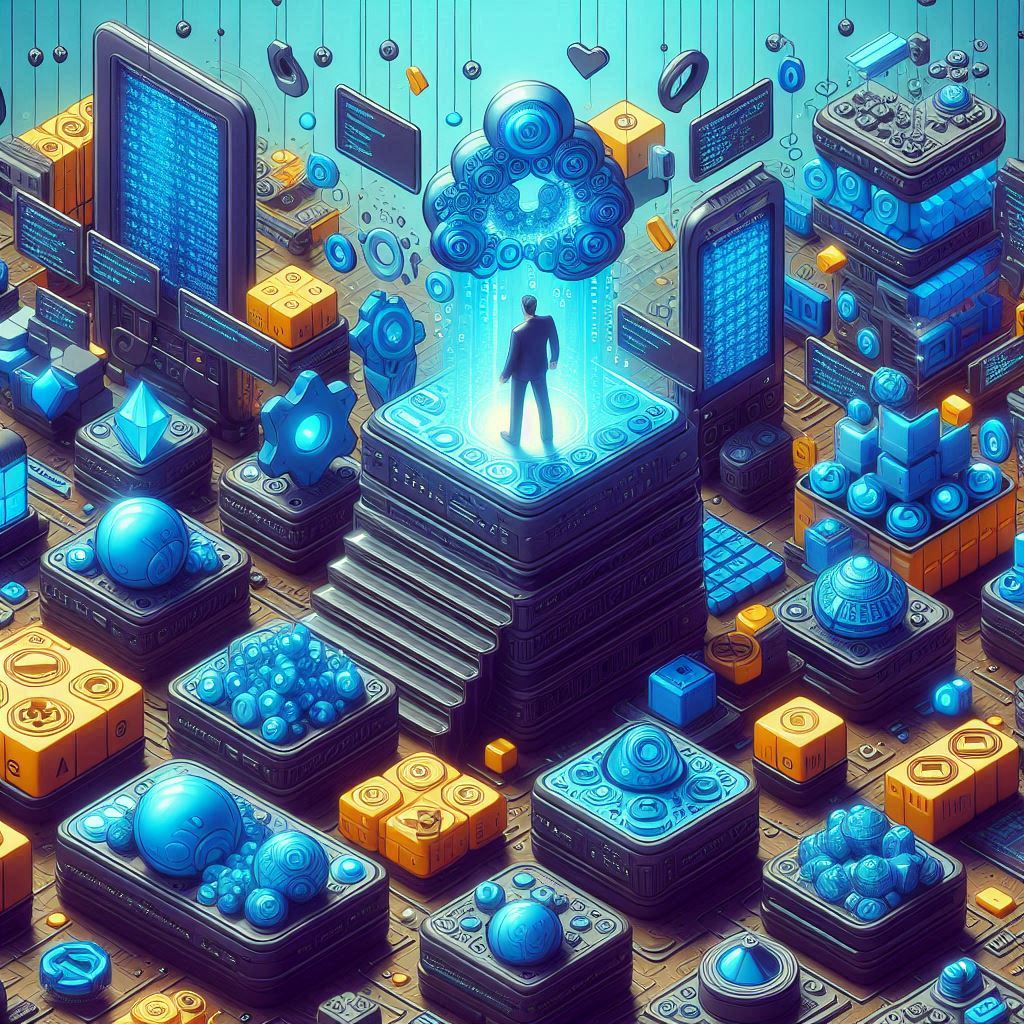
The Problem We’re Solving
Picture this: You’re working on a user management system, dealing with multiple data sources. You need to:
- Remove duplicate user records
- Find users who exist in system A but not in system B
- Identify overlapping user groups
- Merge user lists while eliminating duplicates
Sure, you could write nested loops and maintain temporary HashSets – but there’s a more elegant way.
Distinct: Your First Line of Defense
The Distinct
operation is deceptively simple yet incredibly powerful. Here’s a real-world scenario I encountered last week:
// Imagine getting this messy data from a legacy system
var userEmails = await GetUserEmailsFromLegacySystem();
var cleanEmails = userEmails.Distinct();
But the real magic happens with DistinctBy
. Recently, I needed to find unique companies in our customer database:
public class Customer
{
public string Name { get; set; }
public string CompanyDomain { get; set; }
public string Department { get; set; }
}
// Get one representative per company
var companyRepresentatives = customers.DistinctBy(c => c.CompanyDomain);
Except: The Difference Detective
Except
is your go-to operation when you need to find items present in one collection but not another. Here’s how I used it in a recent data migration project:
// Finding users who haven't converted to the new system
var legacyUsers = await GetLegacyUsers();
var convertedUsers = await GetConvertedUsers();
var usersToMigrate = legacyUsers.ExceptBy(
convertedUsers.Select(u => u.Email),
lu => lu.Email
);
Intersect: Finding Common Ground
Last month, I was working on a feature to identify users who belong to multiple groups. Intersect
made this trivial:
// Finding premium users who are also beta testers
var premiumSubscribers = await GetPremiumSubscribers();
var betaTesters = await GetBetaTesters();
var premiumBetaTesters = premiumSubscribers.IntersectBy(
betaTesters.Select(bt => bt.UserId),
ps => ps.UserId
);
Union: Bringing It All Together
Union
is perfect for merging collections while automatically handling duplicates. Here’s a real example from an analytics dashboard I built:
// Combining active users from multiple services
var webAppUsers = await GetWebAppUsers();
var mobileAppUsers = await GetMobileAppUsers();
var apiUsers = await GetApiUsers();
var allUniqueUsers = webAppUsers
.Union(mobileAppUsers)
.Union(apiUsers);
Performance Considerations
A quick note on performance: While these operations are convenient, they do have O(n) space complexity as they need to maintain hash sets internally. For small to medium collections, the clarity they bring to your code far outweighs any performance overhead. For very large collections, consider using HashSet<T> directly if performance is critical.
My Favorite Pattern: The Pipeline Approach
Here’s a pattern I use frequently that combines multiple set operations:
public async Task<IEnumerable<User>> GetTargetedUsers(
DateTime campaignDate)
{
var activeUsers = await GetActiveUsers();
var blacklistedUsers = await GetBlacklistedUsers();
var previouslyContacted = await GetPreviouslyContactedUsers(campaignDate);
return activeUsers
.Except(blacklistedUsers)
.Except(previouslyContacted)
.DistinctBy(u => u.Email);
}
This pattern is readable, maintainable, and handles all edge cases elegantly.
In Conclusion
LINQ set operations have become an indispensable part of my C# toolkit. They’ve helped me write cleaner, more maintainable code and solve complex business problems with elegant solutions. The next time you find yourself writing nested loops to handle collection operations remember these powerful LINQ methods.
This entire coding session is covered in YouTube Video here.
If you like this blog, please check out this blog on LINQ Partitioning Operators.
What’s your favorite LINQ operation? Have you used these in interesting ways? Let me know in the comments below!